Develog2. Programming Architecture
Hello!✨
Starting from this blog, I’ll be introducing how our team overcame coding-related challenges throughout this project, along with the solutions we developed. At the same time, I’ll also be keeping a personal work log, documenting the specific problems I worked on, the decisions I made, and the features I contributed to.
This series will serve both as a technical breakdown of our development process and a detailed record of my own journey as a developer.
Introduction
As part of our recent development goals, we aimed to implement a system that allows smooth switching between turn-based (Board Mode) and real-time (Arena Mode) gameplay. Since the game requires both strategic planning and action-based combat, having two distinct modes, and we needed a reliable way to switch between them.
How to Switch Between Game Modes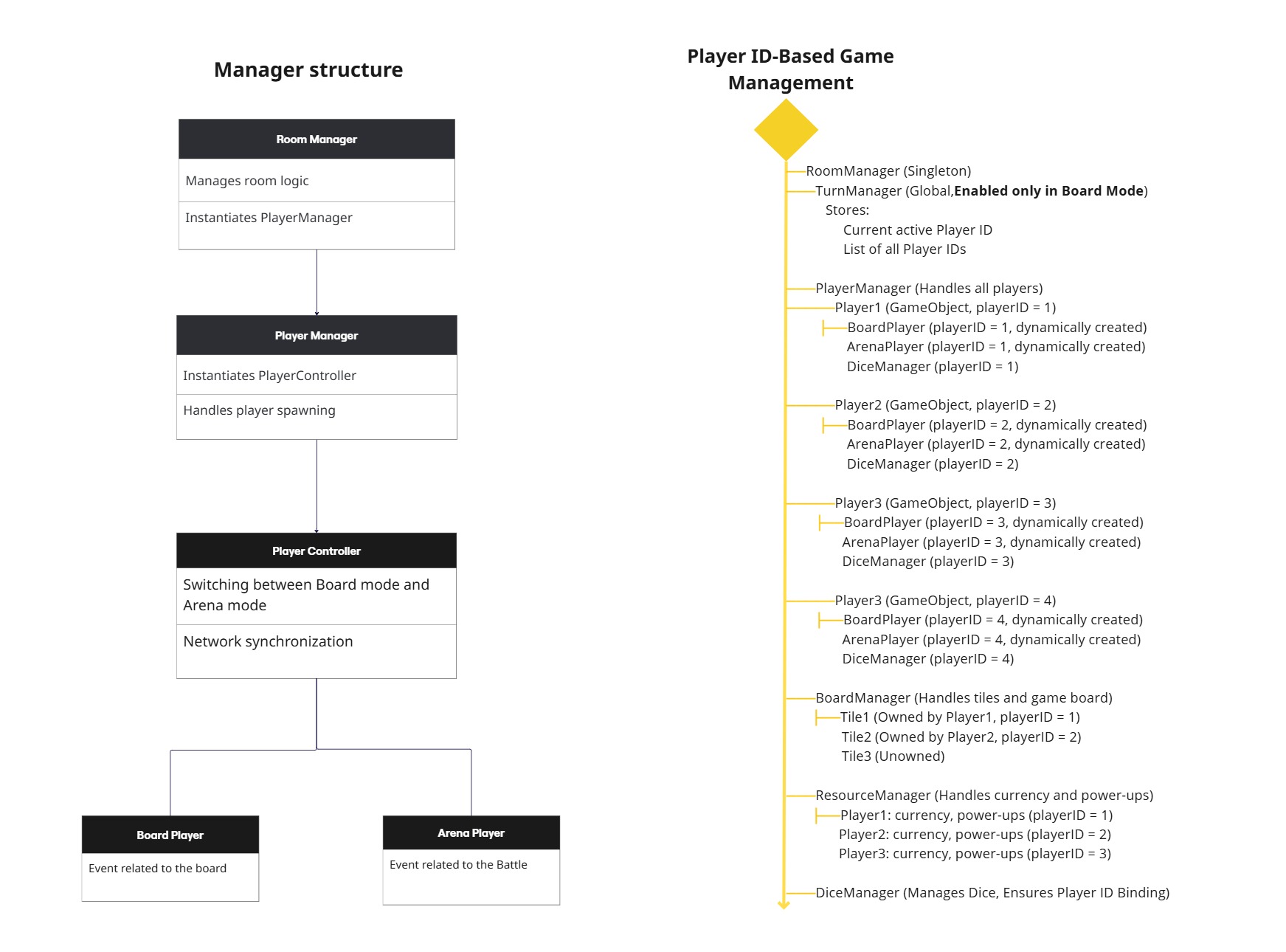
Programming architecture diagram
Mode Switching Logic
One of the key features in our gameplay is enable switching by enabling/disabling different character prefabs depending on the current game phase. The PlayerController held both BoardPlayer and ArenaPlayer objects. During gameplay, we toggle these two by enabling one and disabling the other based on events. For example, when a player triggers the battle event, PlayerController disables BoardPlayer and activates ArenaPlayer. After combat, it switches back. All transitions are synchronized across the network using PhotonView.
Manager Scripts
RoomManager.cs ← Instantiates player manager into main game scene
PlayerManager.cs ← Instantiates player controller into main game scene
PlayerController.cs ←contains both kind of player prefabs
- BoardPlayer.cs ← Player logic – turn-based movement, tile interaction, and event triggering.
- ArenaPlayer.cs ← Player logic– real-time combat with actions like Attack().
Problems I Encountered
Initially several issues showed up during testing:
- Network Desynchronization: Enabling and disabling objects only happened locally, causing clients to fall out of sync. One player could be in combat mode while another still sees them in board mode.
- Lack of Ownership Control: Multiple clients were able to trigger mode switches for the same player, leading to conflicting state changes.
How I Solved It
-
Network-Safe Mode Switching
All mode changes are triggered through [PunRPC] functions, ensuring all clients update simultaneously. Also I added Photon View and Photon Transfrom View for both BoardPlayer and ArenaPlayer to make sure the scene synchronization. -
Exclusive Client Authority
Ensure that only the owning client (verified with PV.IsMine) can initiate the switch. So it can prevent other players from affecting someone else’s game state.
Details
Room Manager:
void OnSceneLoaded(Scene scene, LoadSceneMode LaodSceneMode)
{
if(scene.buildIndex == 2) // replace with the correct index
{
PhotonNetwork.Instantiate(Path.Combine("PhotonPrefabs", "PlayerManager"), Vector3.zero, Quaternion.identity);
}
}
- Manages room logic and ensures Singleton pattern implementation.
- Instantiates PlayerManager when specific scenes are loaded.
Player Manager:
void Start()
{
if (PV.IsMine)
{
CreateController();
}
}
void CreateController()
{
playerController = PhotonNetwork.Instantiate(Path.Combine("PhotonPrefabs", "PlayerController"), Vector3.zero, Quaternion.identity);
playerController.SetActive(true);
}
- Handles instantiating the PlayerController dynamically when a player joins.
- Ensures that logic executes only on the local client using PV.IsMine to check ownership.
- Manages player respawning by destroying and recreating the PlayerController.
PlayerController:
[PunRPC]
private void SwitchToArenaMode()
{
if (boardPlayer != null && arenaPlayer != null)
{
boardPlayer.SetActive(false);
arenaPlayer.SetActive(true);
Debug.Log($"{PV.Owner.NickName} (ID: {photonView.ViewID}) Swtich to Arena mode");
}
}
[PunRPC]
private void SwitchToBoardMode()
{
if (boardPlayer != null && arenaPlayer != null)
{
arenaPlayer.SetActive(false);
boardPlayer.SetActive(true);
Debug.Log($"{PV.Owner.NickName} (ID: {photonView.ViewID}) Swtich to Board mode");
}
}
- Controls switching between BoardPlayer mode (turn-based) and ArenaPlayer mode (real-time combat).
- Implements network synchronization via PhotonView, ensuring all clients receive updates.
Snippet of running game with 2 players
As shown in the image above, each player in the scene has their own instance of both a PlayerManager and a PlayerController. The PlayerController contains two child objects: BoardPlayer and ArenaPlayer, which means all mode-related logic is encapsulated within the player's own controller.
Dice & Domination
A high-stakes multiplayer strategy game with domination and combat
Status | In development |
Author | Angir |
Genre | Strategy |
Tags | 3D, Board Game, monopoly, Multiplayer, Top down shooter |
Languages | English |
More posts
- Devlog1. Game Design of Dice&Domination13 days ago
Leave a comment
Log in with itch.io to leave a comment.