Devlog3. Multiplayer Board Mechanics I
How to do Multiplayer Board Mechanics
Challenges
In an online multiplayer board game, resource and turn management can be difficult. Unlike local games that operate on a single device, multiplayer games must maintain synchronization across all clients. This requirement introduces several common issues:
- Unclear ownership: If the game does not clearly define ownership, multiple players may attempt to roll dice or purchase the same tile simultaneously.
- Desynchronization of turns: If each client manages turns independently, players may fall out of sync. This can result in broken game logic or unfair advantages.
Solution
To address these problems, we decided to use a Player ID-based management method. It means each player has a unique Player ID, which is used to manage:
- Dice (Handled by DiceManager, ensuring dice rolls are bound to the correct Player ID).
- Resources (Currency, power-ups, property, etc.).
- Board interactions (Movement, tile purchases, and events).
e.g.
TurnManager (Global Turn Management)
The TurnManager is created to manage turns across all players. It is only active in Board Mode and is not needed in Arena Mode. It uses Player IDs to track whose turn it is
TurnManager Workflow:
- When a player's turn begins, TurnManager notifies their BoardPlayer to enable movement.
- All other players remain in a waiting state and cannot act.
- Once the active player completes their actions, the turn automatically switches to the next player.
Dice Rolling and Board Movement System
In Dice & Domination, players roll a physical-looking dice and move across a looping board made of tiles.
System Structure
- DiceSide.cs : Check which side of the dice is facing up after rolling.
- DiceManager.cs : Rolls the dice, detects the final value, and synchronizes the result over the network.
- TilesRoute.cs: Holds the ordered list of tiles that players move across.
- BoardPlayer.cs: Moves the player according to dice results and triggers landing events.
Implementation
DiceSide:
The DiceSide class detects which face of the dice is touching the ground after it finishes rolling. Each side collider checks if it is resting on a surface tagged as "BattleGround". If a side touches the ground, it updates its onGround status and used later to determine which number was rolled.
As part of the setup, each dice side's Side Value matches the number of dots shown, and the opposite sides are matched logically (1 is opposite 6, 2 is opposite 5, 3 is opposite 4).
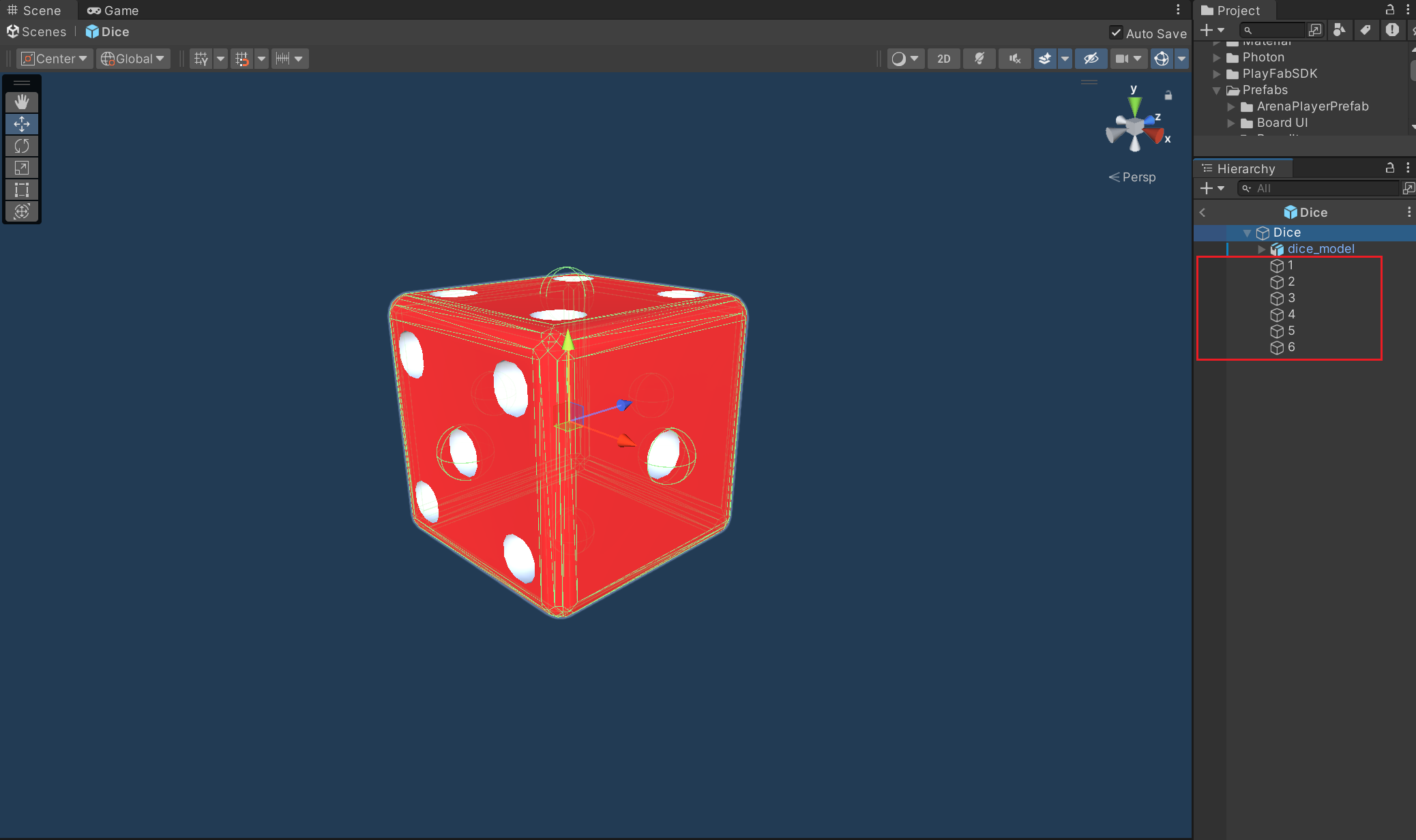
Six colliders on the dice surface
Set Side Value "1" on the surface 6
DiceManager:
This class handles rolling the dice and syncing the roll across all players in a multiplayer session. When the player clicks the "Roll Dice" button, it triggers a roll only if the dice hasn’t already been thrown. It applies a random torque to the dice, making it spin physically. It also sends the torque values to other players using Photon RPC, so all players see the same roll. ‘
In Update() , it monitors when the dice stops moving using Rigidbody.IsSleeping(). When the dice has landed, it freezes its physics, checks each dice side and finds which number is facing up
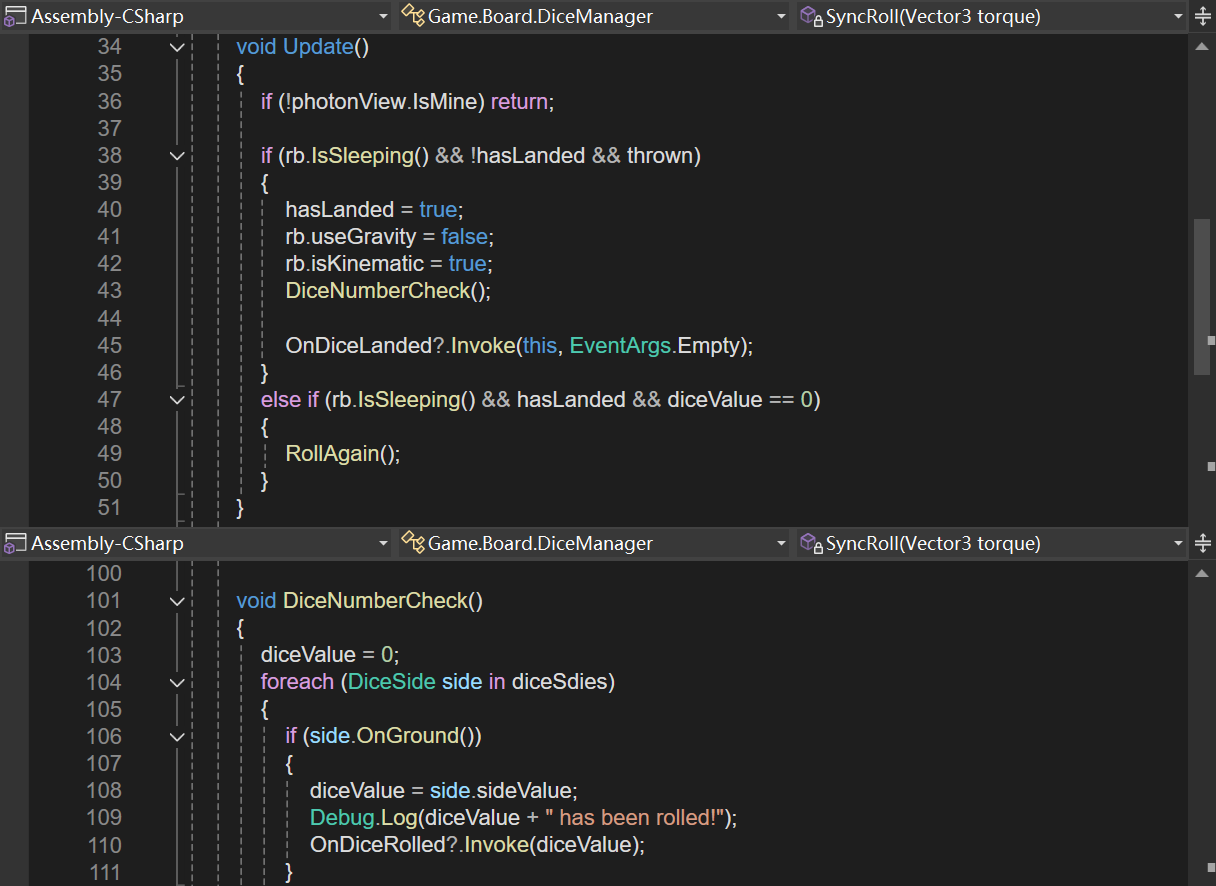
TilesRoute:
The TilesRoute class organizes the path players move along.It automatically gathers all child tiles into a list called childTileList.
BoardPlayer:
The BoardPlayer class controls each player’s movement across the board after rolling the dice. When a dice roll is completed, it listens to the OnDiceRolled event and starts moving.
In MoveOnBoard(), the player advances one tile at a time for each number rolled. In the while loop, it keeps track of the player's current tile index RoutePosition, and after each step, it updates the position based on the board’s tile list. If the player’s movement wraps around the board (meaning the player passes the start tile at index 0), the game detects this by comparing the previous tile index and the new one. When this happens, it rewards the player with extra money. The MoveToNextTile() method moves the player’s position toward the next tile.
After finishing the move, it checks which tile the player landed on and shows the tile information UI or triggers events like battles or purchasement.
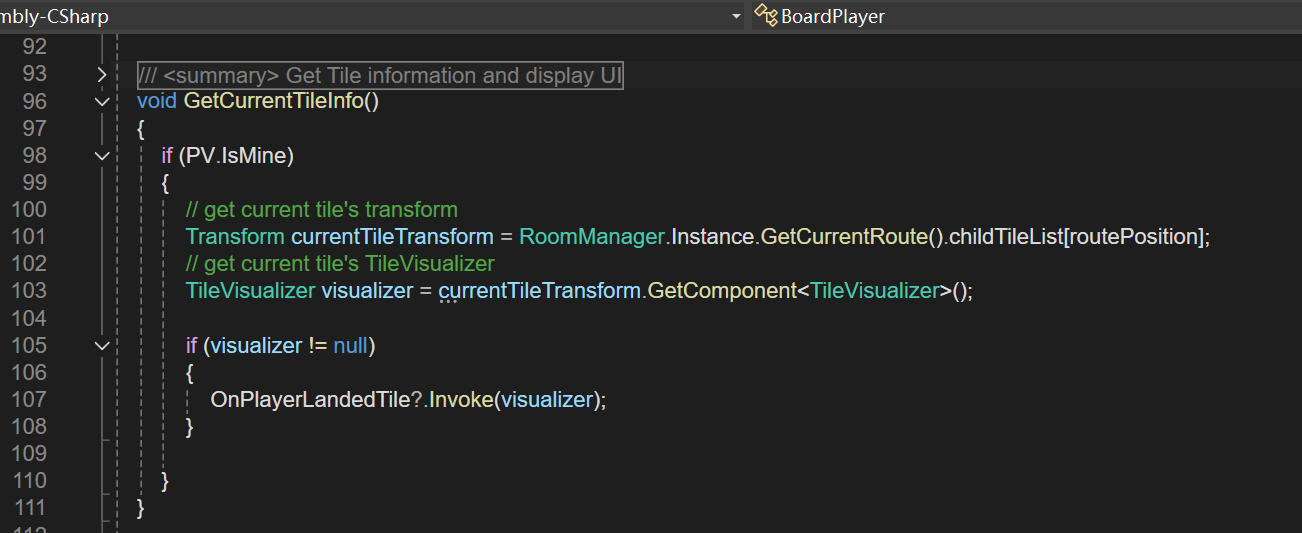
Gameplay Demonstration
Initial setup of dice rolling and route movement
Final effect of dice rolling and route movement
Dice & Domination
A high-stakes multiplayer strategy game with domination and combat
Status | In development |
Author | Angir |
Genre | Strategy |
Tags | 3D, Board Game, monopoly, Multiplayer, Top down shooter |
Languages | English |
More posts
- Develog4. Multiplayer Board Mechanics II1 day ago
- Devlog2. Programming Architecture28 days ago
- Devlog1. Game Design of Dice&Domination37 days ago
Leave a comment
Log in with itch.io to leave a comment.