Devlog6. UI Display of Dice&Domination
Introduction
As the game’s tile system became data-driven and multiplayer-ready, we also needed a UI system that could reflect those properties in real time. The goal was to build a set of flexible and reusable UI panels that could display tile data, enable player interactions (like buying or paying rent), and support multiplayer syncing. This system includes three core UI components and one manager script. Together, they connect closely with the TileVisualizer, TileCSVReader, and other gameplay systems.
System Structure
- TileUIManager.cs: handles UI logic based on tile state, whether it’s owned by others, for sale, or owned by the player.
- TileInfoUI.cs: displays detailed tile information including price, rent, color, and owner,etc.
- SellingPanelUI.cs: shows a scrollable list of owned tiles, allowing players to view or sell them.
- PlayerListUI.cs: displays player names, money, clan icons, and a turn highlights.
Each of these components uses data from TileVisualizer, which in turn pulls from TilesData loaded by TileCSVReader. All visual changes are triggered by gameplay events like landing on a tile or purchasing one.
Implementation
TileInfoUI:
When a player lands on a tile, TileUIManager receives the tile instance and passes it to TileInfoUI, which then updates the on-screen text,owner, and color values.
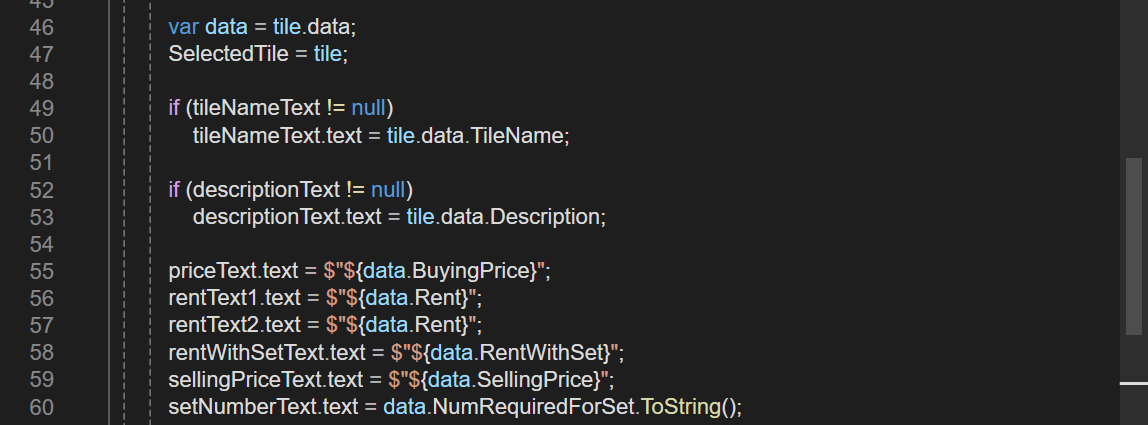
Finding tile owner:
int ownerId = tile.ownerPlayerId; Player ownerPlayer = PhotonNetwork.CurrentRoom?.GetPlayer(ownerId); ownerText.text = ownerPlayer != null ? ownerPlayer.NickName : "Unknown";
TileUIManager:
UI states change depending on tile ownership. For example, if the tile is unowned, a "Not Owned " panel shows. If it’s owned by another player, a “Owned by others” panel appears. And if it's the local player’s own tile, a “Welcome home” panel is shown instead. These panels are toggled with SetActive() based on ownership flags.
The Buy button is only enabled if the tile is for sale and the player has enough money. This is determined by checking the local player's balance and comparing it to the tile price. When a player passes the starting tile, a reward panel popup appears on screen.
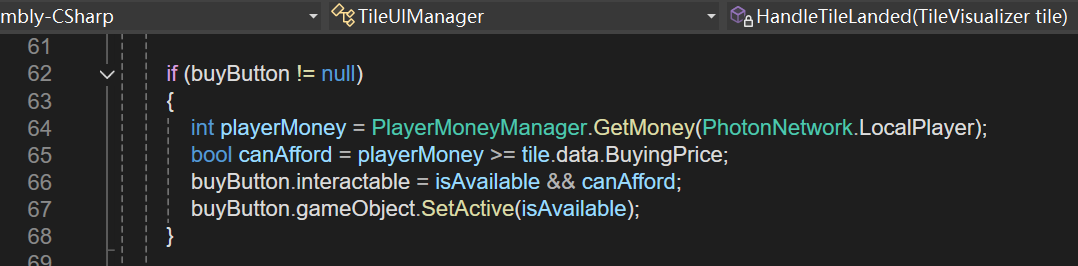
To support different interaction scenarios, I built modular UI panels that can be shown or hidden depending on context. Whether a tile is available for purchase, already owned, or triggering a rent event, the same UI canvas can display different combinations of panels. This makes the system flexible and easy to extend later.
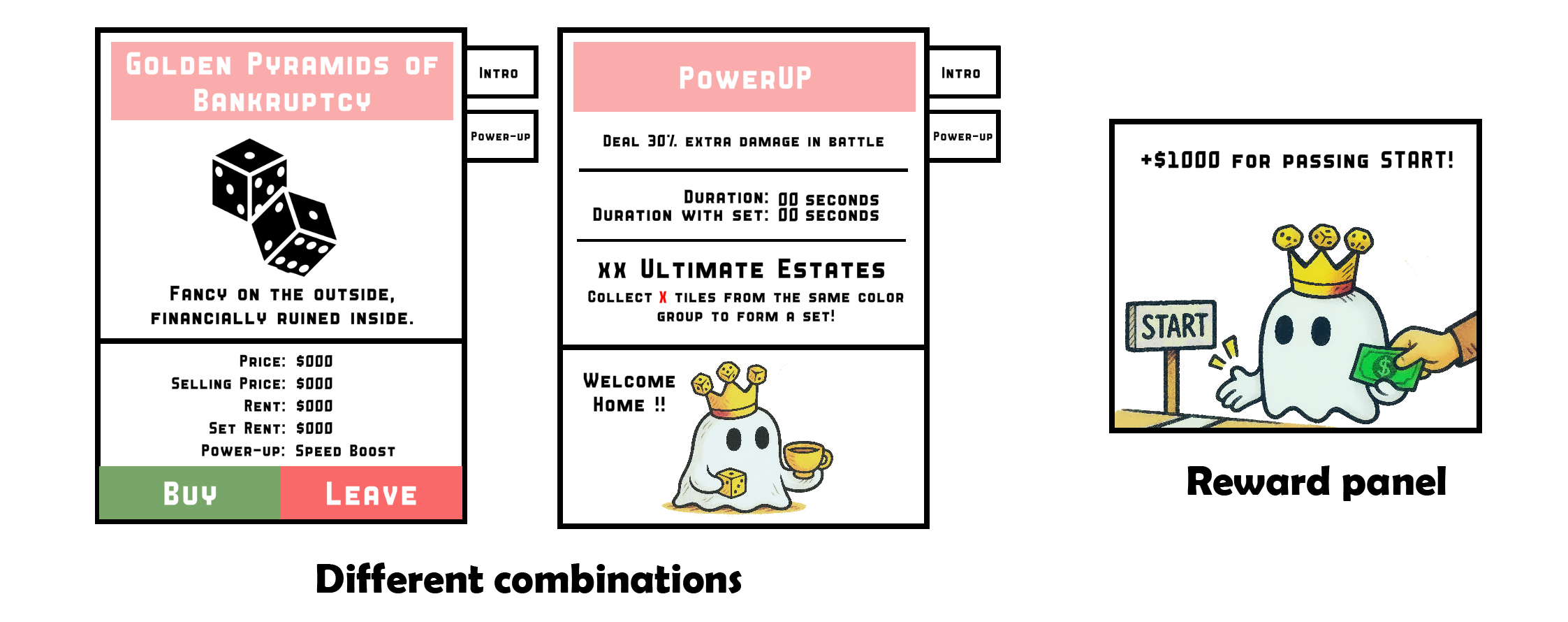
SellingPanelUI:
It creates a scrollable list of the player’s owned tiles using a prefab bar for each entry. These entries are color-coded, clickable, and show the tile name. When clicked, the panel reuses TileInfoUI to display details.
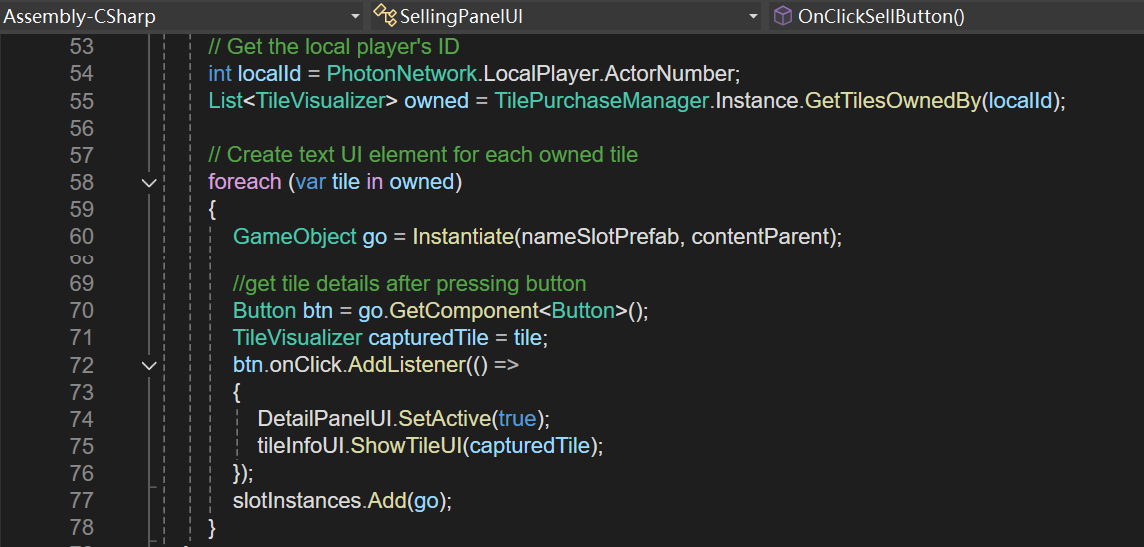
PlayerListUI:
PlayerListUI displays each player's name, money, and icon. When a player's balance changes, a Photon callback triggers a visual update. A small popup animates the amount gained or lost beside their slot.
Gameplay Demonstration
Tile UI display
Tile property list( Selling list)
Final Outcome and Analysis
The UI system now correctly responds to tile interaction events during gameplay. This system can support future expansion with reusable UI elements and shared data flow. Because the panels are modular and context-driven, they can be reused in other phases of the game without duplication.
Design Patterns
- Mediator: TileUIManager acts as a central hub that decides which UI panel to show based on game state, without direct coupling between gameplay logic and UI layout.
- Observer: UI elements subscribe to gameplay events like OnPlayerLandedTile, OnTilePurchased, and update automatically when those events are triggered.
- State: Different UI panels (e.g. owned, unowned, self-owned) represent different visual states of the same canvas, switched by game logic.
Reference
I took UI inspiration from the game Kiospoly. I captured reference shots and stored them in PureRef to guide layout, which became helpful for color design during development:
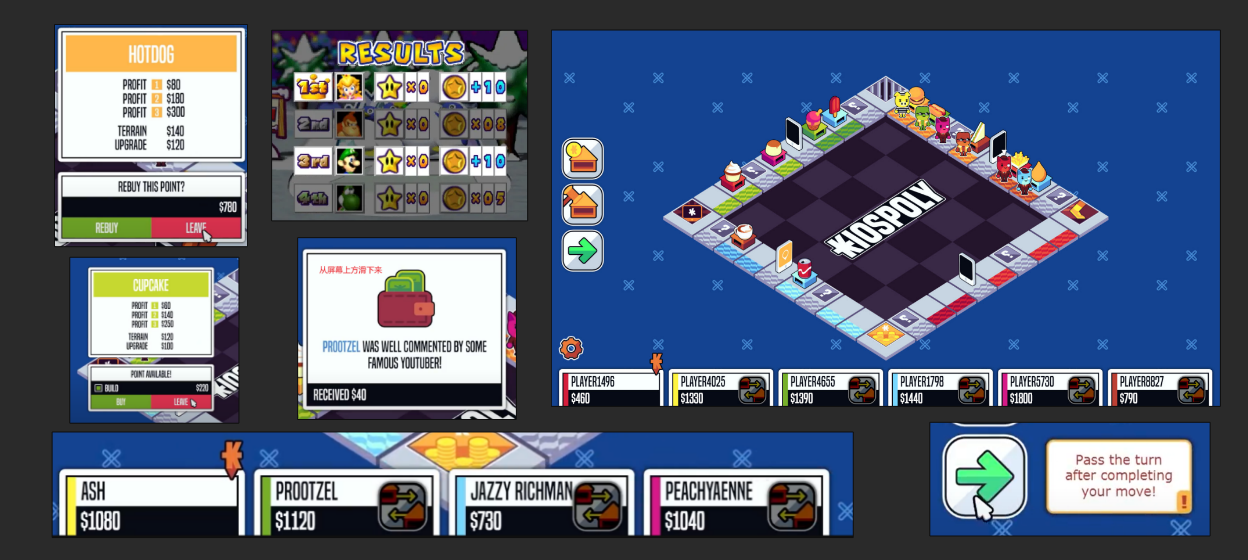
- [1] Photon Engine. (n.d.). Multiplayer Game Development Made Easy. Available at: https://www.photonengine.com/ [Accessed 23 Apr 2025].
- [2] Miro, 2025. https://miro.com/app/dashboard/ [Accessed 23 Apr 2025].
- [3]GameJolt (2022) Kiospoly by Kioskman. [online] Available at: https://gamejolt.com/games/kiospoly/700699 [Accessed 23 Apr 2025].
- [4]PureRef (n.d.) PureRef – The simple way to view and organize your reference images. [online] Available at: https://www.pureref.com/ [Accessed 23 Apr. 2025].
- [5]zanatlija, D., 2022. Pocoyo TV Font [font]. FontSpace. Available at: https://www.fontspace.com/pocoyo-tv-font-f122580 [Accessed 23 April 2025].
Dice & Domination
A high-stakes multiplayer strategy game with domination and combat
Status | In development |
Author | Angir |
Genre | Strategy |
Tags | 3D, Board Game, monopoly, Multiplayer, Top down shooter |
Languages | English |
More posts
- Devlog9. Mode Switching System12 hours ago
- Devlog8. Backend System and Camera Setup1 day ago
- Devlog7. Money and Tile Ownership1 day ago
- Devlog5. Turn Management4 days ago
- Devlog4. Designing Scalable Board Data6 days ago
- Devlog3. Dice Rolling and Board Movement8 days ago
- Devlog2. Programming Architecture33 days ago
- Devlog1. Game Design of Dice&Domination42 days ago
Leave a comment
Log in with itch.io to leave a comment.